Introduction
Recently, I had a requirement to fill the database with some data. Filling the tables manually proved to be very tedious enough. So, I was looking for some software that could do the heavy lifting for me and also that could be used with Python natively. Voila!! After a bit of search came across this awesome software.
FAKER
Faker can be used to generate fake data which resembles the real world values rather than some gibberish values.
Using FAKER With Python
The following code has been tested using Python 3.5 on a Windows 10 machine.
Type the below command in the Command Prompt to install Faker module.
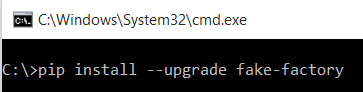
Let’s start with a very simple example.
Import the faker module and make use of the Factory Class. Use create() method to create an object called fake. Say, if you want to print a name then use the object fake along with name() method as shown below.
from faker import Factory
fake = Factory.create()
print(fake.name())
Output
Susan Craig
You can even localize the names according to your preferences.
For example, if you want to display name in Hindi (India), then pass the string argument “hi_IN” to create() method.
from faker import Factory
fake = Factory.create("hi_IN")
print(fake.name())
Output
रामशर्मा, निशा
If you want to display 10 random currencies
from faker import Factory
fake = Factory.create()
for i in range(10):
print(fake.currency_code())
Output
IDR
TZS
SZL
TZS
JOD
MZN
RSD
HUF
SZL
USD
You can randomly generate addresses using address() method. Below code is localized for “en_US”
.
from faker import Factory
fake = Factory.create("en_US")
for i in range(10):
print(fake.address())
Output
84510 Cindy Trail Suite 830
South Jennifer, NC 08359
Unit 7559 Box 9529
DPO AE 67015
502 Thomas Points Apt. 560
Villarrealfurt, KY 87444
31098 Davis Road Suite 959
Conniemouth, NV 35490
918 Mark Lakes
East Amyborough, IN 18369
4382 Shelly Run Suite 212
South Stevenmouth, OH 60501
29782 Karen Turnpike Apt. 373
Underwoodside, PA 50883
415 Andrews Canyon
Kerrstad, AK 38784
5889 Michael Prairie Apt. 678
Masonchester, TX 67688
0629 Warren Islands
Fullerburgh, DE 75136
You can generate various information about a person using different methods as shown below.
from faker import Factory
fake = Factory.create("en_US")
print(fake.last_name_male())
print(fake.name_female())
print(fake.prefix_male())
print(fake.prefix())
print(fake.name())
print(fake.suffix_female())
print(fake.name_male())
print(fake.first_name())
print(fake.suffix_male())
print(fake.suffix())
print(fake.first_name_male())
print(fake.first_name_female())
print(fake.last_name_female())
print(fake.last_name())
print(fake.prefix_female())
King
Natasha Oliver
Mr.
Mr.
Michael Conley
MD
James Ferrell
Stacy
DVM
MD
Paul
Danielle
Cook
Taylor
Dr.
You can read various methods available in Faker Module in detail @
https://faker.readthedocs.io/en/master/#
You can find the above code @
Comments
comments powered by Disqus